Quick Start with building multilingual classifier by power of OpenAI in 5 minutes
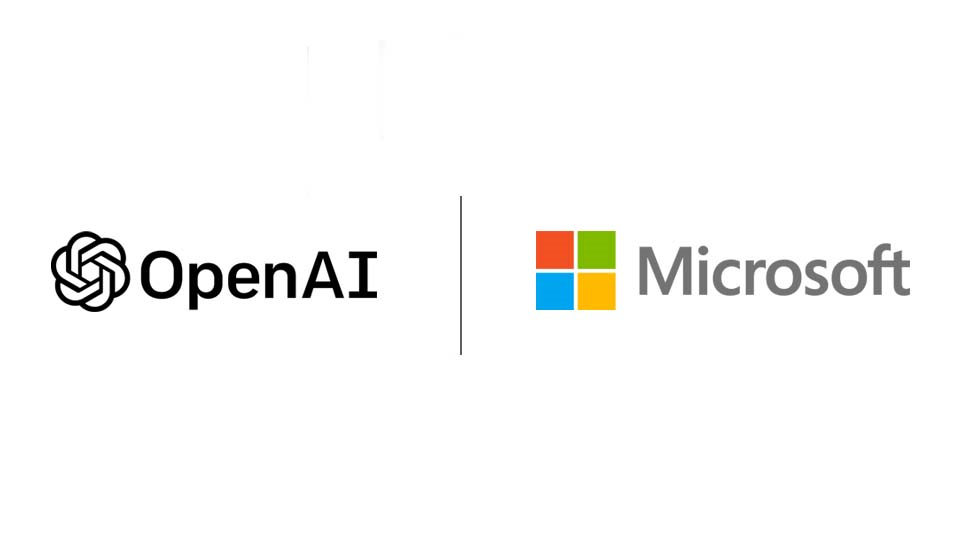
Contents
image: https://blogs.microsoft.com/blog/2023/01/23/microsoftandopenaiextendpartnership/
What is Openai ChatCompletion
- OpenAI Chat API, you can build your own applications with
gpt-3.5-turbo
andgpt-4
Prompt Engineering Overview
- Completion
- Given the unfinished text, complete the text by the GPT model
- Zero shot
- Ask the model to generate the text without any prompt example
- One shot
- Give one sample before giving the assignment
- Few shot
- Give a few samples before giving the assignment
- Chain of thought
- Require model to generate the process with inference steps
- Role prompting / Instruction Tuning
- Bring in the role to awake model’s functionality
Reference
Pricing
- One token is approximately 0.75 word
- 0.002 USD per 1000 tokens
Reference
Quick Start with ChatGPT
Installation
|
|
Create python script
|
|
Making the classifier with few-shot learning and instruction tuning
In this example, I will use the gpt-35-turbo
model to build a sentiment classifier to classify the text into positive
, neutral
or negative
to inference the person is going to work or not.
I use the following concept:
Few-shot learning
Instruction tuning
FAQ
- Q: What’s the difference between openai and azure-openai
- A: Azure-openai provide the service monitoring for model usage but they both use openai client library
- Q: How to set the request timeout in openai client library?
- A: You can set it in the
create
function
|
|
- Q: Can I specify the api information in the create method?
- A: Yes
|
|